Box And Whisker Chart Example using JFreeChart

This Example shows you how to create a box and whisker chart using JFreeChart.
Code of the chart given below shows product value at different date .
In the code given below we have extended class ApplicationFrame to create a frame and also pass a string value to the constructor of ApplicationFrame class by using super keyword that will be name of the created frame.
Methods used in this example are described below:
pack(): This method invokes the layout manager.
centerFrameOnScreen(): This method is used for the position of the frame in the middle of the screen.
setVisible(): This method is used for display frame on the screen.
add(): This method is used for added data into DefaultBoxAndWhiskerXYDataset class object.
createBoxAndWhiskerChart(): This method is used to create box and whisker chart for given values. It takes title, domain axisa label,
range axis label, dataset and legend as parameters.
BoxAndWhiskerChart.java
import java.awt.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.statistics.BoxAndWhiskerCalculator;
import org.jfree.data.statistics.BoxAndWhiskerXYDataset;
import org.jfree.data.statistics.DefaultBoxAndWhiskerXYDataset;
import org.jfree.date.DateUtilities;
import org.jfree.ui.ApplicationFrame;
import org.jfree.ui.RefineryUtilities;
public class BoxAndWhiskerChart extends ApplicationFrame {
public BoxAndWhiskerChart(String titel) {
super(titel);
final BoxAndWhiskerXYDataset dataset = createDataset();
final JFreeChart chart = createChart(dataset);
final ChartPanel chartPanel = new ChartPanel(chart);
chartPanel.setPreferredSize(new java.awt.Dimension(500, 300));
setContentPane(chartPanel);
}
private BoxAndWhiskerXYDataset createDataset() {
final int ENTITY_COUNT = 14;
DefaultBoxAndWhiskerXYDataset dataset = new
DefaultBoxAndWhiskerXYDataset("Test");
for (int i = 0; i < ENTITY_COUNT; i++) {
Date date = DateUtilities.createDate(2003, 7, i + 1, 12, 0);
List values = new ArrayList();
for (int j = 0; j < 10; j++) {
values.add(new Double(10.0 + Math.random() * 10.0));
values.add(new Double(13.0 + Math.random() * 4.0));
}
dataset.add(date,
BoxAndWhiskerCalculator.calculateBoxAndWhiskerStatistics(values));
}
return dataset;
}
private JFreeChart createChart(
final BoxAndWhiskerXYDataset dataset) {
JFreeChart chart = ChartFactory.createBoxAndWhiskerChart(
"Box and Whisker Chart", "Time", "Value", dataset, true);
chart.setBackgroundPaint(new Color(249, 231, 236));
return chart;
}
public static void main(final String[] args) {
final BoxAndWhiskerChart demo = new BoxAndWhiskerChart("");
demo.pack();
RefineryUtilities.centerFrameOnScreen(demo);
demo.setVisible(true);
}
}
|
Output:
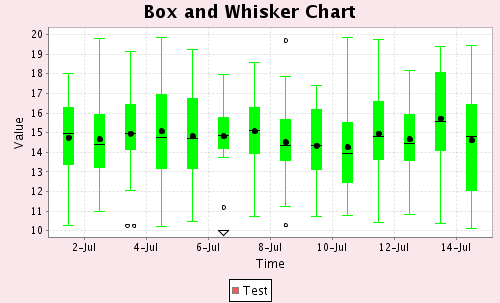
Download
code