Create area chart in JSP page using JFreeChart
This Example shows you how to create a area chart in JSP page using JFreeChart. Code given below creates a area chart of runs of two teams in different matches. In the code given below we have extended class ApplicationFrame to create a frame and also pass a string value to the constructor of ApplicationFrame class by using super keyword that will be name of the created frame.Methods used in this example are described below:
pack(): This method invokes the layout manager.
centerFrameOnScreen(): This method is used for the position of the frame in the middle of the screen.
setVisible(): This method is used for display frame on the screen.
createCategoryDataset(): This method is used to create the instance of CategoryDataset Interface and that contains a copy of the data in an array.
createAreaChart(): This method is used to create bar chart for given values. It takes title, category axis label, value axis label, dataset, Plot Orientation, legend, tool tips and urls as parameters.
saveChartAsPNG(): This method is used to save chart in to png format.
jspareachart.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
|
Output:
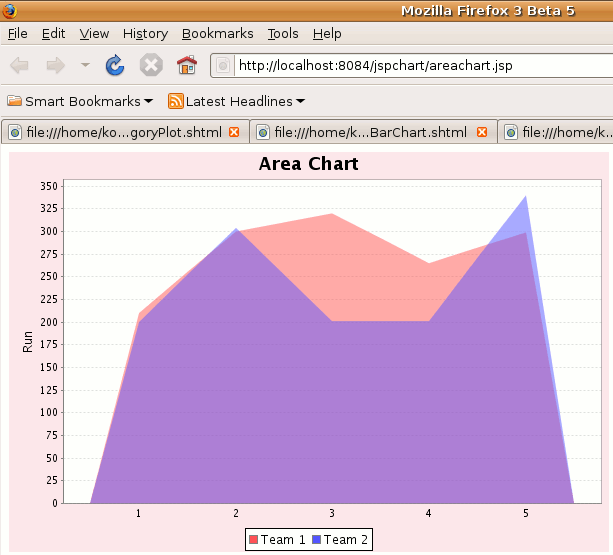
Download code