Bar Chart Example using JFreeChart
This Example shows you how to create a bar chart using JFreeChart.Code given below creates a bar chart of scores of two teams in matches.
Description of the code:
In this code we have extended class ApplicationFrame to create a frame and also pass a string value to the constructor of ApplicationFrame class by using super keyword that will be name of the created frame.
Some methods that are used in this code :
pack(): This method invokes the layout manager.
centerFrameOnScreen(): This method is used for the position of the frame in the middle of the screen.
setVisible(): This method is used for display frame on the screen.
createCategoryDataset(): This method is used to create the instance of CategoryDataset Interface and that contains a copy of the data in an array.
createBarChart(): This method is used to create bar chart for given values. It takestitle, category axis label, value axis label, dataset, PlotOrientation, legend, tool tips and urls as parameters.
BarChartDemo1.java:
|
Output:
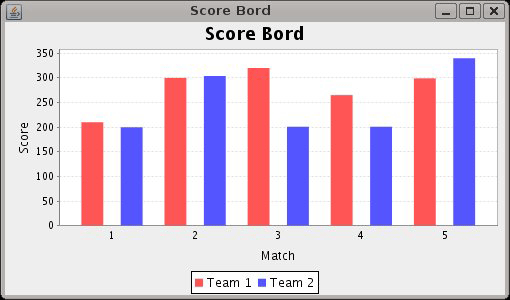
Download code