Demonstrates TableTree

This section shows you Table Tree.
SWT provides the class TableTree to display the hierarchy of items in
a tabular form. In the given example, we have defined three columns for the
table tree. The method getTable() of class TableTree returns
the underlying table control . The method table.setHeaderVisible(true)
makes the header visible. The class TableColumn creates the columns in
the TableTree. The class TableTreeItem adds the items to the TableTree.
Here is the code of TableTreeExample.java
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.*;
import org.eclipse.swt.layout.*;
import org.eclipse.swt.widgets.*;
public class TableTreeExample {
static int COL = 3;
private static void createContents(final Shell shell) {
shell.setLayout(new FillLayout());
TableTree tableTree = new TableTree(shell, SWT.NONE);
Table table = tableTree.getTable();
table.setHeaderVisible(true);
for (int i = 0; i < COL; i++) {
new TableColumn(table, SWT.LEFT).setText("Column " + (i + 1));
}
for (int i = 0; i < COL; i++) {
TableTreeItem parent = new TableTreeItem(tableTree, SWT.NONE);
parent.setText(0, "Book " + (i + 1));
parent.setText(1, "Core Java");
parent.setText(2, "Advanced Java");
for (int j = 0; j < COL; j++) {
TableTreeItem child = new TableTreeItem(parent, SWT.NONE);
child.setText(0, "Java " + (j + 1));
child.setText(1, "Core Java Topics");
child.setText(2, "Advanced Java Topics");
}
parent.setExpanded(true);
}
TableColumn[] tableColumn = table.getColumns();
for (int i = 0, n = tableColumn.length; i < n; i++) {
tableColumn[i].pack();
}
}
public static void main(String[] args) {
TableTreeExample example= new TableTreeExample();
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("TableTree");
createContents(shell);
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
|
Output will be displayed as:
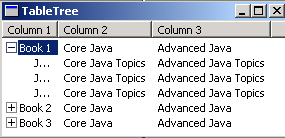
Download Source Code
