Create a ToolBar

This section illustrates you how to create a ToolBar in SWT.
To create a ToolBar , we have used the class ToolBar. The method setBounds()
sets the size and location of toolBar. The class ToolItem adds the item
to the ToolBar. The SWT.PUSH creates the toolItem in the form of
button on the toolBar. The method setText() of this class sets the text
to the toolItem. We have used the Listener class to display the selected toolItem
on the console.
Here is the code of ToolBarExample.java
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.layout.FillLayout;
public class ToolBarExample {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("Toolbar");
shell.setLayout(new FillLayout());
createToolbar(shell);
shell.setSize(300,150);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
public static void createToolbar(Shell shell) {
ToolBar toolBar = new ToolBar(shell, SWT.HORIZONTAL);
toolBar.setBounds(5, 5, 350, 30);
ToolItem item1 = new ToolItem(toolBar, SWT.PUSH);
item1.setText("New");
ToolItem item2 = new ToolItem(toolBar, SWT.PUSH);
item2.setText("Open");
ToolItem item3 = new ToolItem(toolBar, SWT.PUSH);
item3.setText("Save");
ToolItem item4 = new ToolItem(toolBar, SWT.PUSH);
item4.setText("Cut");
ToolItem item5 = new ToolItem(toolBar, SWT.PUSH);
item5.setText("Copy");
ToolItem item6 = new ToolItem(toolBar, SWT.PUSH);
item6.setText("Paste");
Listener listener = new Listener() {
public void handleEvent(Event event) {
ToolItem item = (ToolItem) event.widget;
String string = item.getText();
if (string.equals("New"))
System.out.println("New");
else if (string.equals("Open"))
System.out.println("Open");
else if (string.equals("Save"))
System.out.println("Save");
else if (string.equals("Cut"))
System.out.println("Cut");
else if (string.equals("Copy"))
System.out.println("Copy");
else if (string.equals("Paste"))
System.out.println("Paste");
}
};
item1.addListener(SWT.Selection, listener);
item2.addListener(SWT.Selection, listener);
item3.addListener(SWT.Selection, listener);
item4.addListener(SWT.Selection, listener);
item5.addListener(SWT.Selection, listener);
item6.addListener(SWT.Selection, listener);
}
}
|
Output will be displayed as:
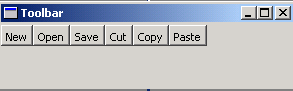
Download Source Code