In this example you will see how to move image smoothly on mouse motion using Java.
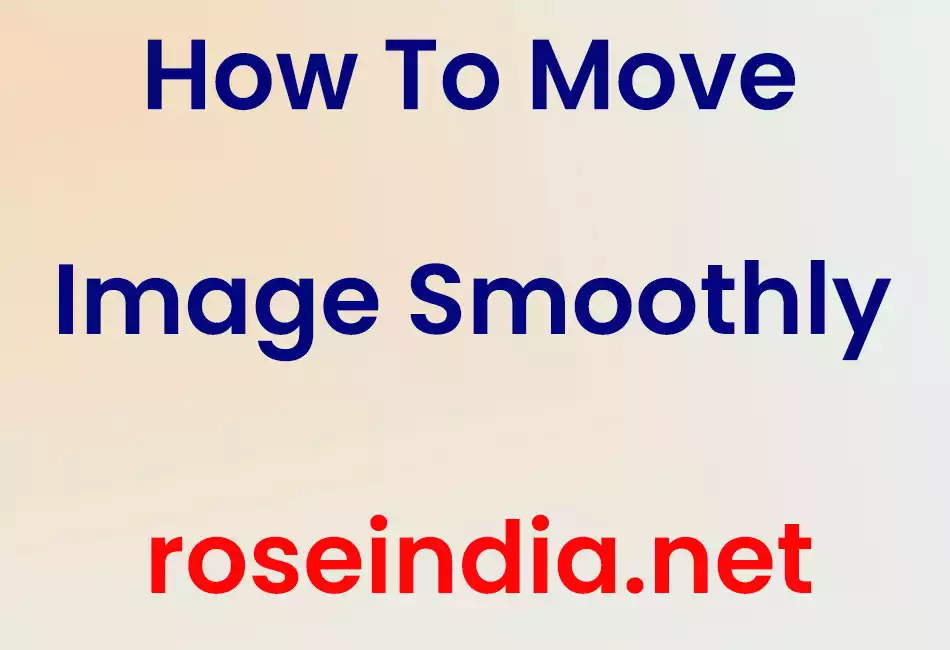
How To Move Image Smoothly

In this example you will see how to move image smoothly on mouse motion using
Java.
To move an image, we have used the Listener interface that provides the mouse motion
events in Java. So in this example you will learn where the MouseMotionListener interface is
called to move an image on mouse motion. In this example we have used the JFrame class of javax.swing.* package
that allows to create frame.
The methods mouseMoved() and mouseDragged() of Listener interface
are defined in the code. The method mouseDragged() is invoked when a mouse button is pressed
on the image. Then the image will dragged. The method mouseMoved() is invoked when
the mouse cursor has been moved on to a image without pushing the image. The
method checkImage() will create the Off-screen image if it has
not been created. This method should always be called before using the
off-screen image. To draw into the offscreen image, paintOffscreen(image.getGraphics())
is called. The g.drawImage(image, 0, 0, null) put the offscreen image on
the screen.
Here is the code of SmoothMoveExample.java
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class SmoothMoveExample extends JPanel implements
MouseMotionListener {
private int X, Y;
private Image image;
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.getContentPane().add(new SmoothMoveExample());
frame.setSize(350, 300);
frame.show();
}
public SmoothMoveExample() {
addMouseMotionListener(this);
setVisible(true);
}
public void mouseMoved(MouseEvent event) {
X
= (int) event.getPoint().getX();
Y = (int) event.getPoint().getY();
repaint();
}
public void mouseDragged(MouseEvent event) {
mouseMoved(event);
}
public void update(Graphics graphics) {
paint(graphics);
}
public void paint(Graphics g) {
Dimension dim = getSize();
checkImage();
Graphics graphics = image.getGraphics();
graphics.setColor(getBackground());
graphics.fillRect(0, 0, dim.width, dim.height);
paintOffscreen(image.getGraphics());
g.drawImage(image, 0, 0, null);
}
private void checkImage() {
Dimension dim = getSize();
if (image == null || image.getWidth(null) != dim.width
|| image.getHeight(null) != dim.height) {
image = createImage(dim.width, dim.height);
}
}
public void paintOffscreen(Graphics g) {
int size = 150;
g.setColor(Color.pink);
g.fillOval(X
- size / 2, Y
- size / 2, size , size );
}
} |
Output will be displayed as:

Download Source Code