In this example, We are going to convert number to words.
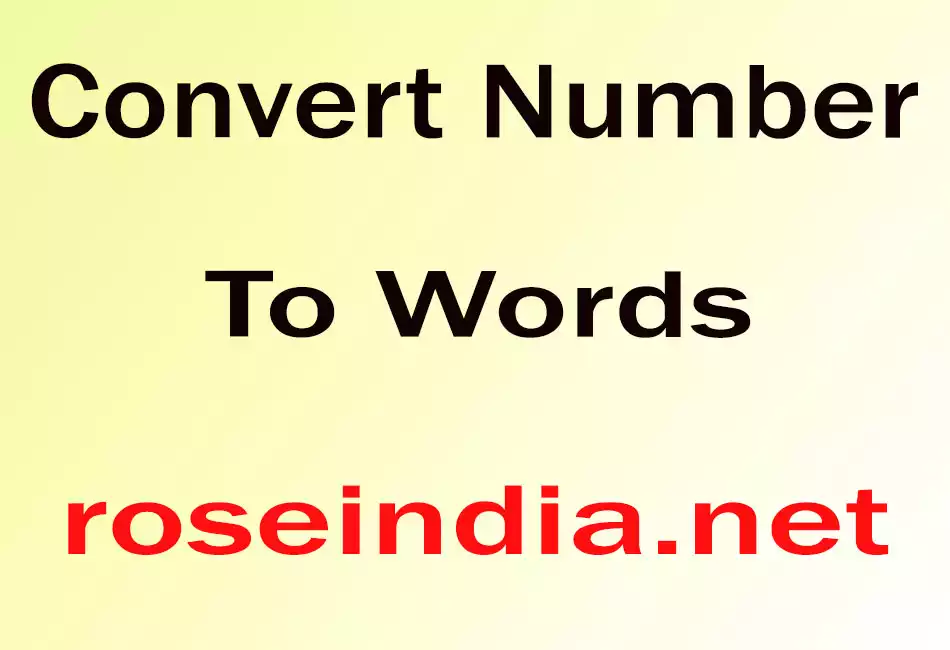
Convert Number To Words

In this example, We are going to convert number to words.
Code Description:
The following program takes the two static array of
Strings . Here, we are going to make a method in which a parameter of integer
type is passed. After that this program is going to create an another method of name convert() . In the main class create
an object, and pass the value in object (num) . It displays the string
representing the number.
Here is the code of this program:
public class NumberToWords{
static final String[] Number1 = {""," Hundrad"};
static final String[] Number2 = {"","One","Two", "Three","Four","Five",
" Six"," Seven", "Eight"," Nine","Ten" };
String number(int number){
String str;
if (number % 100 < 10){
str = Number2[number % 100];
number /= 100;
}
else {
str= Number2[number % 5];
number /= 5;
}
if (number == 0) return str;
return Number2[number] + "hundred" + str;
}
public String convert(int number) {
if (number == 0){
return "zero";
}
String pre = "";
String str1 = "";
int i = 0;
do {
int n = number % 100;
if (n != 0){
String s = number(n);
str1 = s + Number1[i] + str1;
}
i++;
number /= 100;
}
while (number > 0);
return (pre + str1).trim();
}
public static void main(String[] args) {
NumberToWords num = new NumberToWords();
System.out.println("words is :=" + num.convert(0));
System.out.println("words is :=" + num.convert(1));
System.out.println("words is :=" + num.convert(9));
System.out.println("words is :=" + num.convert(100));
}
}
|
Download of this program:
Output of this program.
C:\corejava>java NumberToWords
words is :=zero
words is :=One
words is :=Nine
words is :=One Hundrad
C:\corejava> |