In JavaScript array we can also detect and remove the duplicate elements by creating a user defined function.
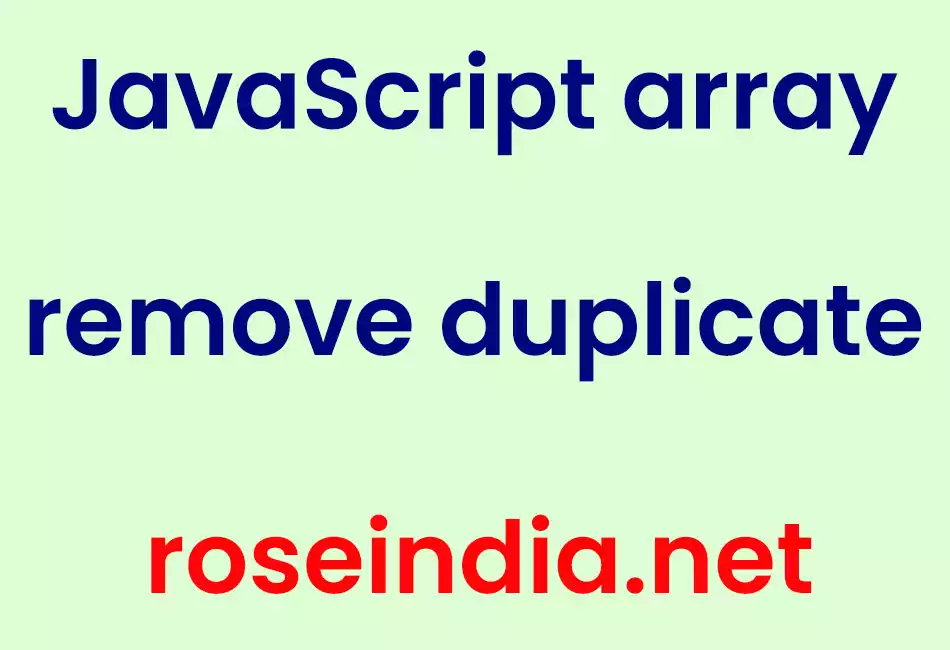
JavaScript array remove duplicate

In JavaScript array we can also detect and remove the
duplicate elements by creating a user defined function. Since there is not any
built-in method in the JavaScript array to remove duplicate elements therefore
we have created a function which will remove all the duplicated elements from
the passed array. Code for the defined function is as given below:
function removeDuplicateElement(arrayName)
{
var newArray=new Array();
label:for(var i=0; i<arrayName.length;i++ )
{
for(var j=0; j<newArray.length;j++ )
{
if(newArray[j]==arrayName[i])
continue label;
}
newArray[newArray.length] = arrayName[i];
}
return newArray;
}
|
In this removeDuplicateElement(arrayName) we
have passed the arrayName (Array Object) at which the operation is to be
performed. Here is the full HTML example code as follows:
javascript_array_remove_duplicate.html
<html>
<head>
<title>
JavaScript array remove duplicate element
</title>
<script type="text/javascript">
var arr = new Array(7);
arr[0]="Rose";
arr[1]="India";
arr[2]="Technologies";
arr[3]="Pvt";
arr[4]="Ltd";
arr[5]="India";
arr[6]="Rose";
function removeDuplicateElement(arrayName)
{
var newArray=new Array();
label:for(var i=0; i<arrayName.length;i++ )
{
for(var j=0; j<newArray.length;j++ )
{
if(newArray[j]==arrayName[i])
continue label;
}
newArray[newArray.length] = arrayName[i];
}
return newArray;
}
document.writeln("Array before calling removeDuplicateElement()
method is =<b>"+arr+"</b></br>");
document.writeln("Array after calling removeDuplicateElement()
method is =<b>"+removeDuplicateElement(arr)+"</b></br>");
</script>
</head>
<body bgcolor="#ddcdff">
<h2>
JavaScript Array Remove duplicate element
</h2>
</body>
</html>
|
Output of example:

Download Sample Code